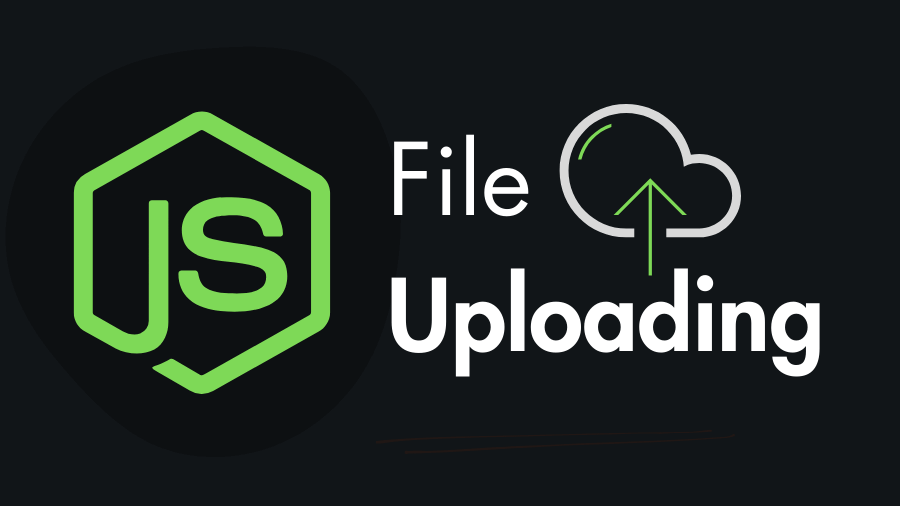
How to Handle File Uploads in Node.js with express-fileupload
File uploads are a key feature in many web applications, and Node.js provides an easy way to manage them. In this tutorial, youβll learn how to upload files to a server using an HTML form and the popular express-fileupload
library.
Step 1: Create a Node.js Project Folder
Start by creating a new project directory and initializing npm:
mkdir file-upload-app
cd file-upload-app
npm init -y
Your folder structure should look like this:
file-upload-app/
βββ app.js # Node.js server file
βββ index.html # HTML file with upload form
βββ uploads/ # Folder where uploaded files will be stored
βββ tmp_folder/ # (Optional) Temporary file storage folder for express-fileupload
βββ node_modules/ # Folder created by npm to store installed packages
βββ package.json # npm configuration file
Step 2: Install Dependencies
Next, install the express
and express-fileupload
packages:
npm install express express-fileupload
Step 3: Create an HTML Form for File Uploads
Create an index.html
file in your projectβs root directory. The form will allow users to select and upload a file. Make sure to add the enctype="multipart/form-data"
attribute to enable file uploads.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Node.js File Upload</title>
<link
rel="stylesheet"
href="https://cdn.jsdelivr.net/npm/water.css@2/out/water.css"
/>
</head>
<body>
<form action="/upload" method="post" enctype="multipart/form-data">
<label for="myFile">Choose a File</label>
<input type="file" name="myfile" id="myFile" />
<input type="submit" value="Upload" />
</form>
</body>
</html>
Step 4: Set Up the Node.js Server
Now, create a file called app.js
to set up your Node.js server. The server will handle file uploads via a POST request to the /upload
route.
const express = require("express");
const fileUpload = require("express-fileupload");
const app = express();
const port = process.env.PORT || 3000;
// Middleware for parsing request bodies
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
// Enable file upload middleware
app.use(fileUpload());
// Serve the HTML form
app.get("/", (req, res) => {
res.sendFile(__dirname + "/index.html");
});
// Handle file uploads
app.post("/upload", (req, res) => {
if (!req.files || Object.keys(req.files).length === 0) {
return res.status(400).send("No files were uploaded.");
}
const targetFile = req.files.myfile;
const uploadPath = __dirname + "/uploads/";
const filePathName = uploadPath + targetFile.name;
targetFile.mv(filePathName, (err) => {
if (err) {
return res.status(500).send(err.message);
}
res.send("File uploaded!");
});
});
// Start the server
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
Step 5: Handle File Upload Errors
You can add error handling to check for missing files or errors during the upload process.
app.post("/upload", async (req, res) => {
try {
const targetFile = req.files.myfile;
await targetFile.mv(__dirname + "/uploads/" + targetFile.name);
res.send("File uploaded!");
} catch (e) {
res.status(500).send(e.message);
}
});
Step 6: Test the Application
To test the upload functionality, run the following command in your terminal:
node app.js
Then, visit http://localhost:3000
in your browser, select a file, and click βUpload.β
Advanced File Upload Features
Here are some additional features you can implement with express-fileupload
:
1. Store Temporary Files in Disk Memory
By default, files are stored in memory. To store them in a temporary file, set the useTempFiles
option:
app.use(
fileUpload({
useTempFiles: true,
tempFileDir: __dirname + "/tmp_folder",
}),
);
2. Limit the Upload File Size
You can limit the size of uploaded files by using the limits
option:
app.use(
fileUpload({
limits: { fileSize: 1 * 1024 * 1024 }, // 1MB max
}),
);
app.post("/upload", (req, res) => {
const targetFile = req.files.myfile;
if (targetFile.truncated) {
return res.send("File is too large. Max size is 1MB.");
}
targetFile.mv(__dirname + "/uploads/" + targetFile.name, (err) => {
if (err) return res.status(500).send(err.message);
res.send("File uploaded!");
});
});
3. Rename the File Before Uploading
To prevent file name collisions, you can rename the file before uploading:
app.post("/upload", (req, res) => {
const targetFile = req.files.myfile;
const newFileName = `${Date.now()}-${targetFile.name}`;
targetFile.mv(__dirname + "/uploads/" + newFileName, (err) => {
if (err) return res.status(500).send(err.message);
res.send("File uploaded!");
});
});
4. Upload Only Specific File Types
You can restrict the file types by checking the file extension:
const path = require("path");
const allowedExtensions = [".png", ".jpg", ".webp"];
app.post("/upload", (req, res) => {
const targetFile = req.files.myfile;
const extension = path.extname(targetFile.name);
if (!allowedExtensions.includes(extension)) {
return res.status(422).send("Invalid file type");
}
targetFile.mv(__dirname + "/uploads/" + targetFile.name, (err) => {
if (err) return res.status(500).send(err.message);
res.send("File uploaded!");
});
});
Youβve successfully set up file uploads in your Node.js application! From here, you can enhance the functionality by adding more features like user authentication and advanced file validation. Happy coding!