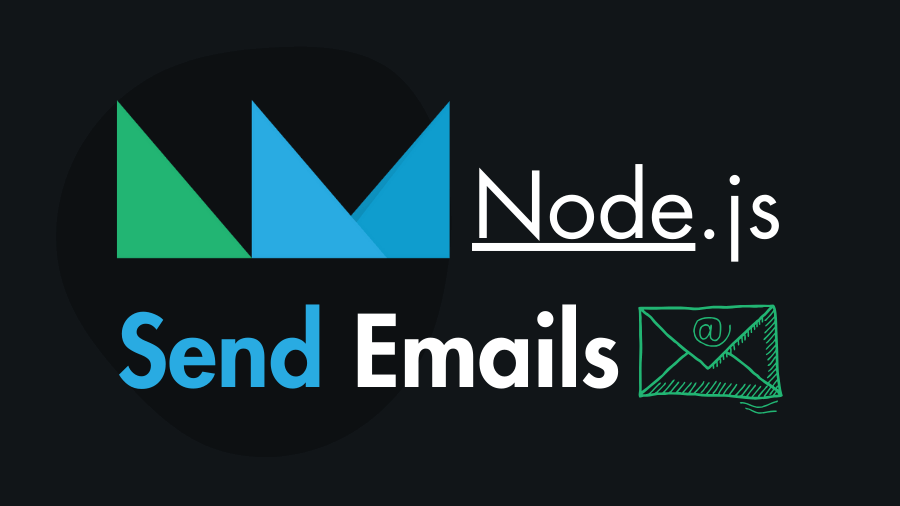
Sending Emails with Attachments in Node.js using Nodemailer
In this tutorial, you’ll learn how to send emails programmatically in Node.js using the Nodemailer library, including sending emails with attachments.
Step 1: Set Up a Node.js Project
First, make sure you have Node.js installed. Then, create a new directory and initialize a new Node.js project:
mkdir node-send-emails
cd node-send-emails
npm init -y
Step 2: Generate a Google App Password for Gmail SMTP
To send emails via Gmail’s SMTP server, you need to generate an app password. Follow these steps:
- Go to Google App Passwords (ensure 2FA is enabled).
- Select an app (e.g., “Mail”) and click Generate.
- Copy the generated password (e.g.,
tnxeizcecpwljdnt
) and save it.
Step 3: Install Nodemailer
Install the Nodemailer package:
npm install nodemailer
Step 4: Configure the Transporter
Create an index.js
file and set up a transporter with Gmail’s SMTP details:
const nodemailer = require("nodemailer");
// Create transporter
const transporter = nodemailer.createTransport({
service: "gmail",
auth: {
user: "[email protected]", // Your Gmail address
pass: "your_app_password", // The app password you generated
},
});
Step 5: Construct the Email Message
Define the email details such as sender, recipient, subject, and message content:
const mailOptions = {
from: "[email protected]", // Sender's email
to: "[email protected]", // Recipient's email
subject: "Hello from Node.js", // Email subject
text: "This is the plain text content.", // Plain text content
html: "<p>This is the <strong>HTML content</strong>.</p>", // HTML content
};
Step 6: Send the Email
Send the email using the transporter:
transporter
.sendMail(mailOptions)
.then((info) => console.log("Email sent:", info.response))
.catch((error) => console.error("Error:", error));
Run your script:
node index.js
Sending Emails to Multiple Recipients
You can send emails to multiple recipients by using an array in the to
or bcc
field:
const mailOptions = {
from: "[email protected]",
to: ["[email protected]", "[email protected]"],
subject: "Hello from Node.js",
text: "Test email sent to multiple recipients!",
};
Adding Attachments to Emails
To send attachments, add an attachments
property to your email options. You can attach files either by providing their content or file paths:
const mailOptions = {
from: "[email protected]",
to: "[email protected]",
subject: "Email with Attachment",
text: "Here is an attachment for you.",
attachments: [
{
filename: "file.txt",
content: "Attachment content as a string or Buffer",
},
{
path: "path/to/file.pdf", // Or provide a file path
},
],
};
Example Code: Sending an Email with an Attachment
Here’s the full example of sending an email with an attachment:
const nodemailer = require("nodemailer");
// Create transporter
const transporter = nodemailer.createTransport({
service: "gmail",
auth: {
user: "[email protected]",
pass: "your_app_password", // Your generated app password
},
});
// Email options with attachment
const mailOptions = {
from: "[email protected]",
to: "[email protected]",
subject: "Email with Attachment",
text: "Here is an attachment.",
attachments: [
{
filename: "file.txt",
content: "Attachment content as a string or Buffer",
},
{
path: "path/to/file.pdf", // Attach a file by path
},
],
};
// Send email
transporter
.sendMail(mailOptions)
.then((info) => console.log("Email sent:", info.response))
.catch((error) => console.error("Error:", error));
👏 Congratulations, you’ve now learned how to send emails using Nodemailer in Node.js, including handling attachments and sending to multiple recipients.