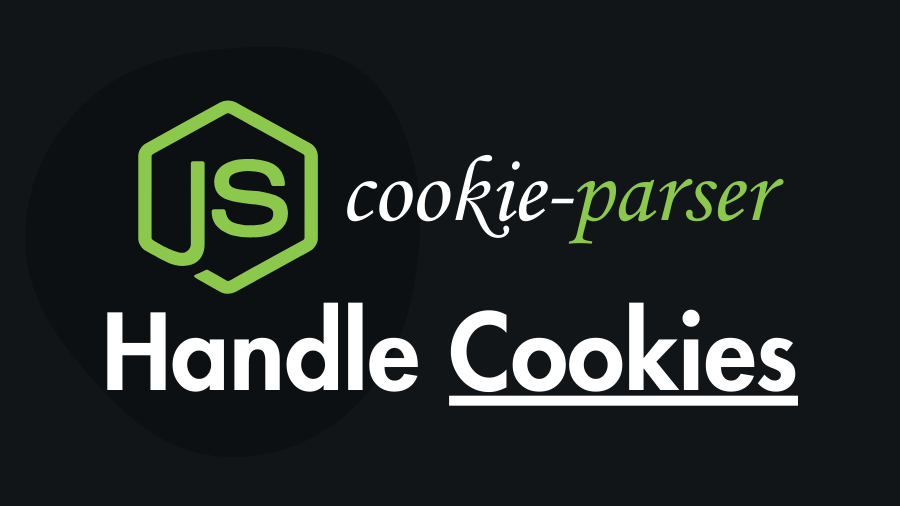
Introduction to Cookies in Node.js with Express
In web development, cookies are essential for maintaining user sessions and storing small pieces of data on the client side. Every time a request is made, cookies are sent along with it to the server. In this tutorial, we’ll show you how to manage cookies in a Node.js application using the Express framework and the cookie-parser middleware.
1. Setting Up a Node.js Project
Before you begin, ensure you have Node.js installed. If you don’t, download and install it from the official website.
Start by creating a new directory for your project and navigate into it in your terminal. Initialize your Node.js project by running:
npm init -y
This command generates a package.json
file with default settings.
2. Installing Express and Cookie-Parser
Next, install the required dependencies—Express and cookie-parser—by running:
npm install express cookie-parser
3. Setting Up the Express Server with Cookie-Parser Middleware
Create a file named app.js
. Inside it, set up an Express server and integrate the cookie-parser
middleware. Here’s the basic setup:
const express = require("express");
const cookieParser = require("cookie-parser");
const app = express();
const PORT = process.env.PORT || 3000;
app.use(cookieParser());
app.listen(PORT, () => console.log(`Server is running on port ${PORT}`));
4. Setting Cookies in Node.js
To set a cookie, use the res.cookie()
method inside a route handler. For example, to set a cookie named username
, you would write:
app.get("/setcookie", (req, res) => {
res.cookie("username", "john", { maxAge: 900000, httpOnly: true });
res.send("Cookie is set");
});
5. Accessing Cookies
To retrieve the cookie value, you can access the cookies via req.cookies
within your route handlers. For example:
app.get("/getcookie", (req, res) => {
const username = req.cookies.username;
res.send("Cookie value: " + username);
});
6. Complete Code for the Express Server
Here is the complete code for your app.js
:
const express = require("express");
const cookieParser = require("cookie-parser");
const app = express();
const PORT = process.env.PORT || 3000;
app.use(cookieParser());
app.get("/setcookie", (req, res) => {
res.cookie("username", "john", { maxAge: 900000, httpOnly: true });
res.send("Cookie is set");
});
app.get("/getcookie", (req, res) => {
const username = req.cookies.username;
res.send("Cookie value: " + username);
});
app.listen(PORT, () => console.log(`Server is running on port ${PORT}`));
7. Running the Server
Start the server by running:
node app.js
Your server will be running on port 3000 by default.
8. Testing Cookie Routes
You can test the cookie routes using a web browser or tools like Postman. Visit /setcookie
to set a cookie and /getcookie
to retrieve its value.
9. Advanced Cookie-Parser Options
Signing Cookies
To prevent cookie tampering, you can sign cookies using a secret key. Here’s how:
const secret = "your-secret-key";
app.use(cookieParser(secret));
Cookie Options
You can specify various options when setting cookies. Some of the common ones are:
- maxAge: The maximum age of the cookie in milliseconds.
- expires: The expiration date of the cookie (Date object).
- httpOnly: Makes the cookie accessible only via HTTP(S).
- secure: Ensures the cookie is sent over HTTPS.
- sameSite: Controls cookie sending in cross-origin requests.
Example:
app.get("/setcookie", (req, res) => {
res.cookie("username", "john", { maxAge: 900000, httpOnly: true });
res.send("Cookie is set");
});
Apply Options Universally
You can also apply options universally to all cookies using cookieParser
like this:
const options = {
maxAge: 900000,
httpOnly: true,
};
app.use(cookieParser(secret, options));