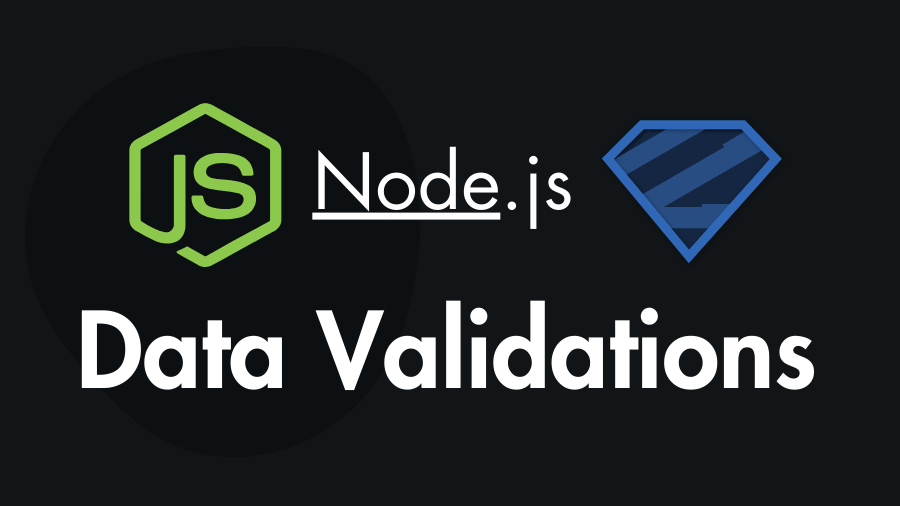
Quick Guide to Data Validation with Zod
Zod is a simple and powerful library for data validation in Node.js and TypeScript. It lets you define rules to ensure your data is correct before processing it. Let’s quickly go through how to use Zod for data validation.
1. Install Zod
First, add Zod to your project with npm:
npm install zod
2. Define a Validation Schema
Zod uses schemas to define validation rules. Here’s how to create one for user data:
const { z } = require("zod");
const userSchema = z.object({
username: z.string().min(3).max(30),
email: z.string().email(),
password: z.string().min(6),
age: z.number().int().min(18),
isAdmin: z.boolean(),
});
In this schema:
username
must be between 3 and 30 characters.email
must be a valid email address.password
must be at least 6 characters.age
must be an integer of 18 or older.isAdmin
must be a boolean.
3. Validate Data
Now, use the schema to validate an object of data:
const data = {
username: "john_doe",
email: "[email protected]",
password: "password123",
age: 25,
isAdmin: false,
};
try {
const validatedData = userSchema.parse(data);
console.log("Valid data:", validatedData);
} catch (error) {
console.error("Validation error:", error.errors);
}
If the data is valid, parse()
returns the validated data. Otherwise, it throws an error with details about the validation failure.
4. Use safeParse
for Error Handling
If you don’t want your app to crash on validation failure, use safeParse
. It returns a result object with either the valid data or an error:
const result = userSchema.safeParse(data);
if (result.success) {
console.log("Valid data:", result.data);
} else {
console.error("Validation failed:", result.error.errors);
}
5. Integrate Zod with Express.js
You can easily use Zod to validate incoming data in an Express app. Here’s a quick example:
const express = require("express");
const { z } = require("zod");
const app = express();
app.use(express.json());
app.post("/user", (req, res) => {
const result = userSchema.safeParse(req.body);
if (result.success) {
res.json({ message: "User created", user: result.data });
} else {
res.status(400).json({ errors: result.error.errors });
}
});
app.listen(3000, () => console.log("Server is running on port 3000"));
In this example, the incoming data is validated before being processed. If the data is invalid, the server responds with validation errors.
6. Zod in React
You can also use Zod on the client side, like in a React form, to validate user input before sending it to the server:
import { useState } from "react";
import { z } from "zod";
const userSchema = z.object({
username: z.string().min(3).max(30),
email: z.string().email(),
password: z.string().min(6),
age: z.number().int().min(18),
isAdmin: z.boolean(),
});
function App() {
const [formData, setFormData] = useState({
username: "",
email: "",
password: "",
age: "",
isAdmin: false,
});
const [errors, setErrors] = useState({});
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
const handleSubmit = (e) => {
e.preventDefault();
const result = userSchema.safeParse(formData);
if (result.success) {
console.log("Valid data:", result.data);
setErrors({});
} else {
setErrors(result.error.flatten());
}
};
return (
<form onSubmit={handleSubmit}>{/* Input fields and error messages */}</form>
);
}
export default App;
Here, safeParse
is used to validate form data. If the data is valid, it’s processed; if not, errors are shown.
Conclusion
Zod makes data validation easy and clean, both in Node.js and React apps. It allows you to define strict rules for your data and handle validation gracefully without complex setups.
For more details, check out the Zod documentation.