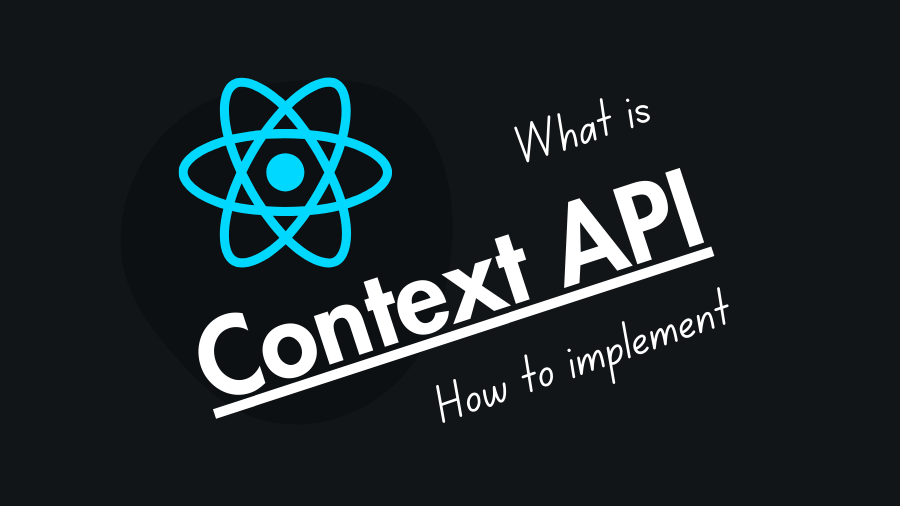
Introduction to the React Context API
The React Context API is a feature that allows you to share state across components without passing props through every level of your component tree. This makes it easier to manage global state, especially in large applications. In this tutorial, you’ll learn how to use the Context API to simplify state management in your React app.
What is the React Context API?
The React Context API, introduced in React 16.3, lets you share state across multiple components without passing props down the component tree. It creates a global context that any component can access, making it especially useful for complex applications or to avoid prop drilling (passing props through many levels).
How to Implement the React Context API
To use the React Context API in your application, follow these steps:
Step 1: Create a Context
First, create a context using createContext()
. The context will act as a container for the shared state.
// MyContext.jsx
import { createContext } from "react";
// Set a default value for the context
const MyContext = createContext("");
export default MyContext;
Here, we create MyContext
with an empty string as the default value. If a component tries to access the context without a provider higher up in the tree, it will use this default value.
Step 2: Define the Context Provider
Next, wrap a portion of your app with the Provider
component. The Provider
makes the context available to all components inside it by passing a value
prop with the data you want to share.
// main.jsx
import React from "react";
import ReactDOM from "react-dom";
import MyContext from "./MyContext";
import MyComponent from "./MyComponent";
const myData = "Hello World!";
ReactDOM.createRoot(document.getElementById("root")).render(
<React.StrictMode>
<MyContext.Provider value={myData}>
<MyComponent />
{/* Other components can be added here */}
</MyContext.Provider>
</React.StrictMode>,
);
Here, the MyComponent
and any other component inside MyContext.Provider
can access the myData
value.
Step 3: Access the Context Data
To access the context data in a component, use the useContext
hook. This provides a simpler, cleaner way to access context compared to the older Consumer
component.
// MyComponent.jsx
import { useContext } from "react";
import MyContext from "./MyContext";
const MyComponent = () => {
const data = useContext(MyContext);
return <div>{data}</div>; // Output: Hello World!
};
export default MyComponent;
In this example, MyComponent
accesses the value provided by MyContext.Provider
and displays it.
Step 4: Update the Context
You can also update the context by passing a new value to the Provider
. React will re-render all components that depend on that context when the value changes.
// App.jsx
import { useState, createContext } from "react";
import Profile from "./Profile";
const AuthContext = createContext("");
const App = () => {
const [user, setUser] = useState("");
return (
<AuthContext.Provider value={{ user, setUser }}>
<Profile />
</AuthContext.Provider>
);
};
export default App;
Now, components like Profile
can access and update the user
data without needing to pass it through props.
// Profile.jsx
import { useContext } from "react";
import { AuthContext } from "./App";
const Profile = () => {
const { user, setUser } = useContext(AuthContext);
return (
<>
<div>Welcome, {user ? user : "Guest"}</div>
<button onClick={() => setUser("React")}>Set User</button>
</>
);
};
export default Profile;
In this example, clicking the button updates the user
state, and all components consuming the context will re-render with the updated value.
Advantages of Using the React Context API
- Simplified Prop Drilling: It eliminates the need to pass props down multiple levels of the component tree, making the code cleaner.
- Global State Management: Context allows you to manage global state that can be accessed by any component in the tree.
- Use of Hooks: The
useContext
hook simplifies accessing context and reduces boilerplate code. - Component Reusability: Components that consume context can be easily reused without worrying about props.
- Default Values: Context provides default values, ensuring that components don’t break when a provider is missing.
Disadvantages of the React Context API
- Complexity for Simple Cases: For small applications or shallow component trees, context might add unnecessary complexity. Simple state management via props or local state might be more appropriate.
- Global State Management: Overusing context for too much state can make the data flow harder to manage and debug.
- Performance Concerns: When context values change, all consumers will re-render. If you have a high frequency of updates or a large component tree, this may impact performance.
Conclusion
The React Context API is a powerful tool for managing shared state in your applications. It simplifies state management by avoiding prop drilling and making data accessible globally. While it’s great for many scenarios, for more complex state management needs, you might consider combining context with other tools like Redux or Zustand.