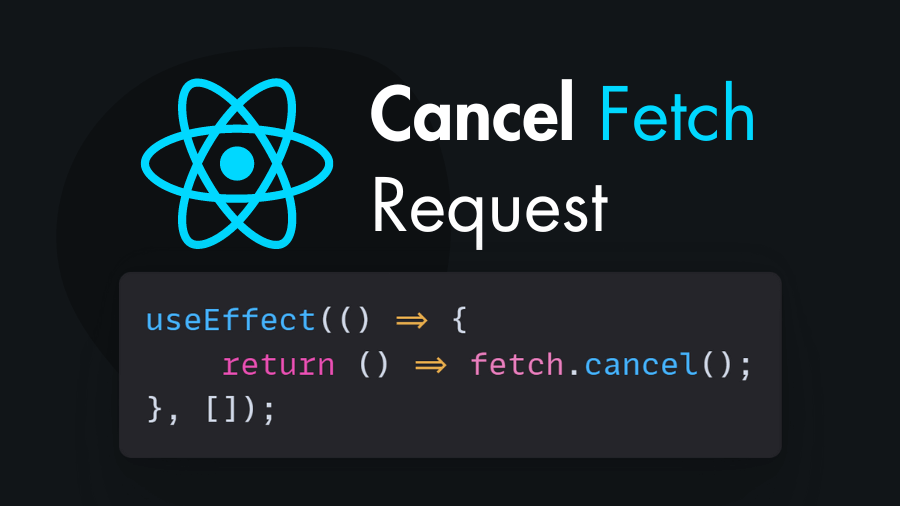
How to Cancel Fetch Requests in React with AbortController
In React.js, fetching data from an API is common. However, you may need to cancel a fetch request if the component unmounts or the user triggers another action before it completes. In this tutorial, you’ll learn how to cancel fetch requests using the AbortController API.
What is the AbortController API?
The AbortController is a built-in web API that lets you cancel asynchronous operations like fetch requests. It creates an instance linked to a signal, and you can call the abort() method to cancel the request.
Why Use AbortController?
- Prevent Memory Leaks: If a fetch request is still in progress when a component unmounts, it may lead to memory leaks or unexpected behavior. Using
AbortController
helps avoid this issue by canceling the fetch request. - Optimize Performance: By canceling unnecessary fetch requests (like when the user navigates away), you reduce unnecessary network activity and improve app performance.
Steps to Implement Fetch Cancellation in React
- Create an
AbortController
instance: This instance is responsible for controlling the fetch request. - Associate the controller with the fetch request: Pass the controller’s signal as part of the fetch options.
- Abort on unmount: When the component unmounts or when you need to cancel the request, call the
abort()
method.
Example Code
import { useEffect, useState } from "react";
const MyComponent = () => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const apiURL = "https://jsonplaceholder.typicode.com/users";
useEffect(() => {
// Create an instance of AbortController
const controller = new AbortController();
const signal = controller.signal;
const fetchData = async () => {
try {
// Fetch data with the signal attached
const response = await fetch(apiURL, { signal });
const result = await response.json();
setData(result);
} catch (error) {
if (error.name === "AbortError") {
console.log("Fetch request was canceled.");
} else {
console.error("Error fetching data:", error);
}
} finally {
// Set loading to false after the request completes (or fails)
setLoading(false);
}
};
fetchData();
// Cleanup: Abort fetch if the component unmounts
return () => controller.abort();
}, []); // Empty dependency array means this effect runs only once on mount
return (
<div>
{loading ? <p>Loading...</p> : <pre>{JSON.stringify(data, null, 2)}</pre>}
</div>
);
};
export default MyComponent;
Explanation:
-
AbortController Instance: We create an
AbortController
and extract itssignal
to pass to the fetch request. -
Fetching Data: In the
fetchData
function, we use thesignal
to cancel the fetch request if needed. If the request is aborted (e.g., when the component unmounts), it throws anAbortError
which we catch and handle separately. -
Cleanup: The
useEffect
hook returns a cleanup function that callscontroller.abort()
. This ensures that the fetch request is canceled if the component unmounts before the request completes. -
Handling Loading State: The loading state (
loading
) is used to show a loading message until the fetch request is complete.
That’s it! You’ve now learned how to cancel fetch requests in React using the AbortController
. This approach helps keep your application efficient and free of unnecessary requests, especially when components are dynamically mounted or unmounted.