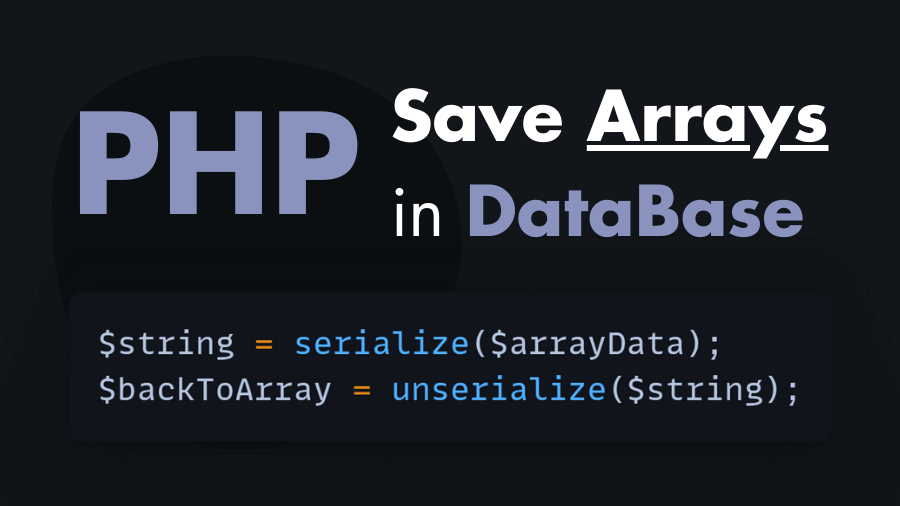
How to Store PHP Arrays in a MySQL Database
If you want to store PHP arrays in a MySQL database, you’re in the right place. This tutorial will show you how to easily store and retrieve PHP arrays using serialization, with practical code examples.
What is Serialization?
Serialization is the process of converting a PHP array into a string format, so it can be safely stored in a database. PHP provides two main functions for this: serialize()
and json_encode()
. Serialization allows you to store complex data structures like arrays while preserving their structure. You can later retrieve and unserialize them back into PHP arrays.
Example: Serializing a PHP Array
Let’s start by serializing a simple PHP array.
<?php
$user = array(
'name' => 'Aakash Chopra',
'age' => 30,
'email' => '[email protected]',
'skills' => array('PHP', 'MySQL', 'JavaScript')
);
// Serialize the array
$serializedData = serialize($user);
echo $serializedData;
?>
Output:
a:4:{s:4:"name";s:13:"Aakash Chopra";s:3:"age";i:30;s:5:"email";s:15:"[email protected]";s:6:"skills";a:3:{i:0;s:3:"PHP";i:1;s:5:"MySQL";i:2;s:10:"JavaScript";}}
This string is a serialized version of the array, ready to be stored in a database.
Storing the Serialized Array in MySQL
To store the serialized array in a MySQL database, you need to:
- Set up a connection to the database.
- Create a table to hold the serialized data.
- Insert the serialized array into the database.
Here’s an example:
<?php
// Sample PHP array
$data = array(
'name' => 'John Doe',
'age' => 30,
'email' => '[email protected]',
'skills' => array('PHP', 'MySQL', 'JavaScript')
);
// Serialize the array
$serializedData = serialize($data);
// Database connection parameters
$servername = "localhost";
$username = "username";
$password = "password";
$database = "my_database";
// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// SQL query to insert serialized data
$sql = "INSERT INTO array_data (serialized_data) VALUES ('$serializedData')";
if ($conn->query($sql) === TRUE) {
echo "Array data stored successfully!";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
// Close the connection
$conn->close();
?>
Retrieving and Unserializing the Array
When you retrieve the serialized data from the database, you can unserialize it back into a PHP array using the unserialize()
function.
Here’s how to retrieve and unserialize the data:
<?php
// Example of serialized data retrieved from the database
$serializedData = 'a:4:{s:4:"name";s:13:"Aakash Chopra";s:3:"age";i:30;s:5:"email";s:15:"[email protected]";s:6:"skills";a:3:{i:0;s:3:"PHP";i:1;s:5:"MySQL";i:2;s:10:"JavaScript";}}';
// Unserialize the data back into an array
$backIntoArray = unserialize($serializedData);
// Print the resulting array
print_r($backIntoArray);
?>
Output:
Array
(
[name] => Aakash Chopra
[age] => 30
[email] => [email protected]
[skills] => Array
(
[0] => PHP
[1] => MySQL
[2] => JavaScript
)
)
Conclusion
Using PHP’s serialize()
and unserialize()
functions, you can easily store and retrieve complex PHP arrays in a MySQL database. Serialization is an effective way to persist array data in databases, allowing you to reconstruct it when needed. Just remember to choose the right column type (like TEXT
or VARCHAR
) in your database to store the serialized data.