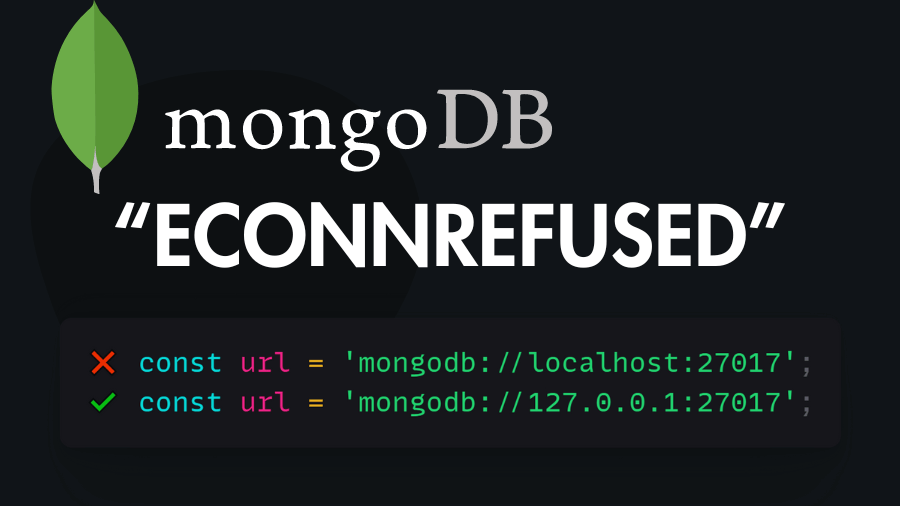
How to Fix the âECONNREFUSEDâ Error in Node.js When Connecting to MongoDB
If youâre seeing the âECONNREFUSEDâ error in your Node.js application, it means that your app is unable to connect to the MongoDB server. This can happen for several reasons, but the good news is that itâs usually easy to fix. In this guide, weâll explain common causes of the error and how to resolve them.
Common Causes of the âECONNREFUSEDâ Error
-
MongoDB Server Not Running
First, make sure your MongoDB server is up and running. If itâs not running, start it with the command:mongod
-
Incorrect MongoDB Connection Settings
Double-check the MongoDB connection settings in your Node.js application. Ensure the server address and database name are correct. Hereâs an example:const { MongoClient } = require("mongodb"); const url = "mongodb://127.0.0.1:27017"; // Make sure this URL is correct const dbName = "mydatabase"; // Make sure this is your actual database name const client = new MongoClient(url);
-
MongoDBâs Bind IP Configuration
By default, MongoDB binds to the local IP address (127.0.0.1), which means it only accepts local connections. If you want to connect from a different machine, youâll need to update the bind IP in MongoDBâs config file (mongod.conf
).Find and update the
bindIp
setting to allow connections from other IP addresses. -
Firewall or Security Software Blocking the Connection
A firewall or security software might be blocking the connection to MongoDB. Ensure that your serverâs firewall allows incoming connections on the default MongoDB port (27017).
Solutions to Fix the âECONNREFUSEDâ Error
-
Use the IP Address Instead of âlocalhostâ
Sometimes, replacing âlocalhostâ with the actual IP address of the server can solve the issue. Update your connection URL in the code like this:// â Instead of this const url = "mongodb://localhost:27017"; // âïž Use this const url = "mongodb://127.0.0.1:27017";
-
Check MongoDBâs Log Files
MongoDB keeps logs that can help identify any issues. If youâre on a Linux system, check the logs in the/var/log/mongodb/
directory. Look for any error messages related to the connection. -
Check for System or Server Restarts
Sometimes, a server or system restart can cause MongoDB to become inaccessible. Ensure that your server is up and running and that MongoDB is correctly started. -
Check for Port Conflicts
MongoDB uses port 27017 by default. If another service is using this port, MongoDB wonât be able to bind to it. Make sure no other service is using port 27017.
Conclusion
By following these steps, you should be able to resolve the âECONNREFUSEDâ error when connecting to MongoDB in your Node.js application. Always ensure MongoDB is running, check your settings, and troubleshoot network or server-related issues.
If the error persists, feel free to consult MongoDBâs documentation or community forums for more help.