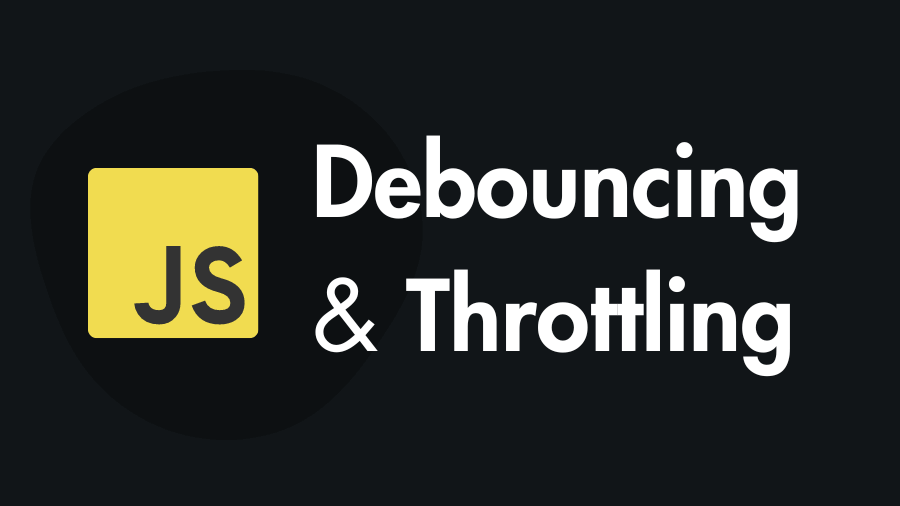
Debounce vs. Throttle: Understanding and Using These Techniques in JavaScript
In JavaScript, debounce and throttle are essential techniques for controlling the frequency of function executions, especially in situations involving high-frequency events like typing, scrolling, or resizing. These techniques help optimize performance and improve the user experience by limiting unnecessary function calls.
In this tutorial, we will explain both techniques, how they work, and when to use each one.
What is Debouncing?
Debouncing is a technique used to delay the execution of a function until a certain amount of time has passed since the last event. It’s useful for handling events that may fire repeatedly, such as typing or scrolling, but you only want to respond to the final event after a pause.
Example of Debouncing:
Imagine you are implementing an auto-complete feature where you want to wait for the user to stop typing before making an API request.
Here’s how you can implement debounce:
function debounce(func, delay) {
let timer;
return function (...args) {
clearTimeout(timer); // Cancel the previous timer if it exists
timer = setTimeout(() => {
func(...args); // Execute the function after the delay
}, delay);
};
}
// Usage: Execute the function after 3 seconds of inactivity
const runAfter3Seconds = debounce(function (name) {
console.log("Hi, " + name);
}, 3000);
runAfter3Seconds("Babu Rao");
When to Use Debounce:
- Auto-complete suggestions: Trigger an action after the user has stopped typing.
- Window resizing or scrolling: Handle these events efficiently without triggering excessive reflows or re-renders.
- Button click events: Prevent multiple rapid clicks on a button that triggers an action.
What is Throttling?
Throttling is a technique that limits how often a function can be executed over a certain period of time. Unlike debouncing, which waits for inactivity, throttling ensures that the function runs at most once per defined time interval.
Example of Throttling:
Let’s say you want to ensure that a function is called at most once every 3 seconds, even if it’s triggered more frequently.
function throttle(func, limit) {
let inThrottle = false; // Flag to track whether the function is in throttle mode
return function (...args) {
if (!inThrottle) {
func(...args); // Call the function
inThrottle = true; // Set the flag to prevent further calls
setTimeout(() => {
inThrottle = false; // Reset the flag after the limit
}, limit);
}
};
}
// Usage: Execute the function at most once every 3 seconds
const sayHi = throttle(function () {
console.log("Hi");
}, 3000);
// Call the throttled function every second (but it will only run once every 3 seconds)
setInterval(() => {
sayHi();
}, 1000);
When to Use Throttle:
- Scroll-based animations: Limit the number of times a scroll event handler fires.
- User interface interactions: Rate-limit actions like dragging, zooming, or resizing to ensure smooth interactions.
- Network requests: Prevent excessive API calls when a user interacts quickly, such as when typing in a search box or navigating pages.
Debounce vs. Throttle: When to Use Each
While both techniques are used to limit function executions, the choice between debounce and throttle depends on the behavior you want to achieve:
-
Use Debounce:
- When you want to ensure that a function is executed only after a period of inactivity.
- Ideal for actions that should happen once, like submitting a form or fetching suggestions in an auto-complete feature.
-
Use Throttle:
- When you need to limit how frequently a function can execute over time.
- Suitable for continuous or repetitive actions, like scrolling, resizing, or animations.
Best Practices
-
Choose the Right Technique:
- Consider whether your scenario requires waiting for inactivity (debounce) or limiting the frequency of execution (throttle).
-
Fine-Tune the Delay or Interval:
- Adjust the delay for debouncing or the time interval for throttling to balance between performance and user experience.
-
Consider User Experience:
- Avoid making the interface sluggish by using overly aggressive debounce or throttle values. Test different values to find the best balance.
-
Test Thoroughly:
- Always test your debouncing or throttling implementation in scenarios with multiple rapid interactions to ensure it works as expected.
By using debounce and throttle techniques correctly, you can significantly enhance the performance of your web applications and improve the user experience, especially when handling high-frequency events.